HOW TO IMPLEMENT DWAVE QBSOLVE IN PYTHON
D-Wave’s Quantum Computer is capable of solving optimization problems in a fraction of the time it would be taken by a classical computer. So what is DWave QBSolve basically in python and how to implement Dwave Qbsolve in Python?
Well, D-Wave’s QBSolv is a tool for solving Quadratic Binary Optimisation problems.
QBSolv is available as a Python library and has been used in a variety of applications.
In this blog, we will guide you step-by-step on how to implement DWave QBsolve in Python. So, Let’s Begin!
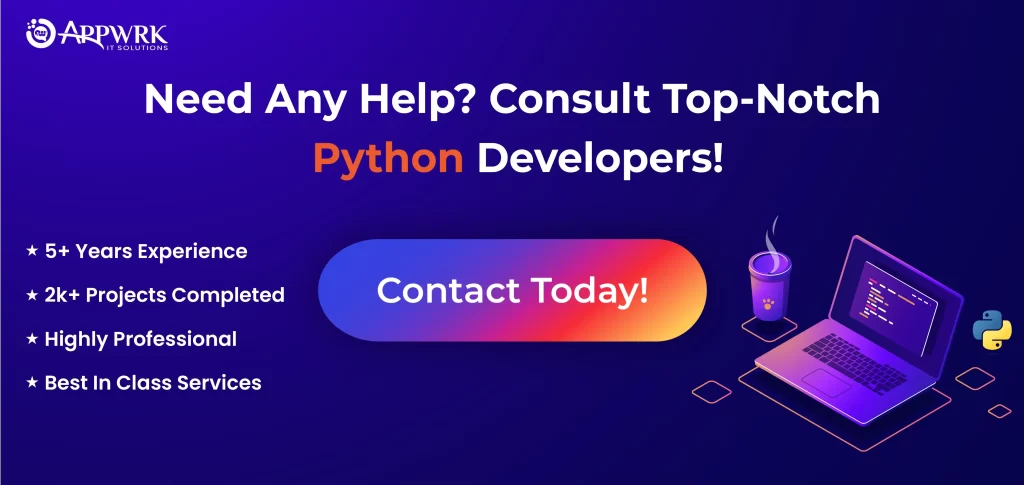
What is the purpose of D-wave QB Solve?
D-wave QB-Solve is a cloud-based service offered by D-wave systems, a Canadian computing company.
The purpose of D-wave QB Solve is to provide users with access to D-wave’s quantum annealing technology, which is designed to solve optimization problems.
Optimization problems are those where the objective is to find the best solution from a set of possible solutions based on certain constraints on criteria.
Quantum Annealing is a specialized technique that uses quantum mechanics to solve optimization problems faster than classical methods.
D-wave QB Solve allows users to submit their optimization problems to D wave’s quantum annealing technology and receive the best possible solution in a short span of time.
D-wave QB-Solve is intended for businesses and researchers who need to solve optimization problems that are difficult or impossible to solve using classical computing methods.
The service is particularly useful for problems that involve large amounts of data or complex mathematical models.
Some examples of applications for D-wave QB-solve include machine learning, logistics, financial Modelling, and drug discovery.
What are the advantages of using Python with QBSolve?
The combination of Python and QB Solve provides developers with a powerful and flexible platform for developing complex optimization algorithms for a wide range of real-world applications.
The ease of use, large ecosystem, and interactivity of Python combined with the power and efficiency of QBSolve make this combination a popular choice among developers and researchers working on optimization problems.
The following are the advantages of using Python with QBSolve:
- Easy to learn: Python is a popular and easy-to-learn programming language.
This makes it easier for developers to start working with QBSolv and quickly implement complex optimization algorithms.
- Large ecosystem: Python has a large ecosystem of libraries and frameworks that can easily be integrated with QBSolv.
This allows developers to leverage existing code and functionality, reducing development time and effort.
- Highly Flexible: Python is a highly flexible language that allows developers to write code in a variety of styles, from procedural to object-oriented.
This makes it easier to write code that is well-organized readable and maintainable.
- Cross-platform language: Python is a cross-platform language meaning that code written on one Operating system can easily run on another.
This makes it easier to develop and deploy QBSolv-based applications across multiple platforms and environments.
- Interactivity: Python is a highly interactive language that allows developers to prototype and test code.
This is especially useful for developing and refining optimization algorithms as it allows developers to see their code in real-time.
Are there any APIs for QBSolve?
QBSolve is a solver in the D-wave ocean Software development Kit, which provides a set of python tools and libraries for solving optimization problems using quantum and classical computing resources.
The ocean SDKs include a number of APIs for interacting with QBSolve and other Solvers including:
- Dimod API: The dimod API is a Python package for defining and manipulating binary quadratic models (BQMs), which are used as input to QBSolv and other solvers.
The dimod package provides a common interface for interacting with various solvers, including QBSolv.
- QBSolv API: The QBSolv API is a Python package that provides a simple interface for calling the QBSolv solver from within a Python program.
The QBSolv API takes a BQM as input and returns the optimal solution to the optimization problem.
- Solver API: The Solver API is a Python package that provides a common interface for interacting with various optimization solvers, including QBSolv.
The Solver API provides a consistent way to submit optimization problems to different solvers and retrieve the results.
In addition to these APIs, the D-Wave Leap quantum cloud service also provides a RESTful API for submitting optimization problems to QBSolv and other solvers hosted on the D-Wave quantum annealing processors.
The Leap API can be accessed from Python using the access library or other HTTP clients.
Implementation of DWave QBsolve in Python
Python is a high-level, interpreted programming language known for its simplicity, ease of use, and versatility.
Python has a clear and concise syntax making it easy for developers to read and write code.
One of the strengths of Python is its extensive standard library which provides a wide range of functionality for tasks such as networking, web development, scientific computing, and more.
It is very evident that qbsolve can be used through the dwave_qbsolv package which provides a python interface to the qbsolve solver.
This package can be installed using Python package manager pip and it provides a convenient way to call the qbsolv solver from Python code.
To implement D-Wave QB-Solve in Python, u can certainly follow the following steps:
1. Install the required packages
You can install these packages using a pip, a package manager for Python. Open your command prompt and run the following command:
pip install dwave-ocean-sdk
2. Define the problem
You can create a problem as a dictionary of variable names and their associated bias and coupling values.
3. Initiate a D-wave Solver
You will need to specify the solver name and the API token that you received from the D-wave leap platform.
4. Solve the problem
The ‘qbsolv()’ function returns a dictionary containing the optimal solution and the associated energy.
Here is an example code snippet that demonstrates how to implement D-Wave QB-Solve in Python:
import dwave.cloud as dc
from dwave.system.samplers import DWaveSampler
from dwave.system.composites import EmbeddingComposite
import dimod
# Define the optimization problem
Q = {(0,0): 1, (1,1): 1, (0,1): -2}
# Instantiate a D-Wave solver
sampler = EmbeddingComposite(DWaveSampler(token='MY_API_TOKEN', solver='MY_SOLVER_NAME'))
# Solve the problem
response = dc.qbsolv.QBSolv().sample_qubo(Q, solver=sampler)
# Print the optimal solution and energy
print("Optimal solution:", response.first.sample)
print("Optimal energy:", response.first.energy)
Note that in the above Code snippet, you will need to replace MY_API_TOKEN and MY_SOLVER_NAME with your own API token and Solver name, respectively.
Real-world Applications of QBSOLV
QBSolv, which is a solver within the D-Wave Ocean SDK, is a classical algorithm designed to solve Ising spin-glass problems and other optimization problems.
QBSolv can be used to solve a wide range of real-world optimization problems, including problems in finance, logistics, and engineering.
Here are some examples of real-world applications of QBSolv:
- Portfolio Optimization One of the most common applications of QBSolv is portfolio optimization, where it can be used to optimize asset allocation strategies for investment portfolios.
QBSolv can be used to find the optimal weights for each asset in the portfolio, based on factors such as expected return, risk, and diversification.
- Supply Chain Management: QBSolv can be used to optimize supply chain management by determining the most efficient distribution of goods and materials.
By minimizing transportation costs and maximizing production efficiency, QBSolv can help companies improve their bottom line.
- Drug Discovery: QBSolv can be used to optimize the discovery of new drugs by modeling the interactions between molecules and predicting their effectiveness in treating specific diseases.
By identifying the most promising drug candidates, QBSolv can help accelerate the drug discovery process and reduce the cost of developing new treatments.
- Traffic Optimization: QBSolv can be used to optimize traffic flow in urban areas by determining the most efficient routes for vehicles to take.
QBSolv can help reduce carbon emissions and improve air quality by minimizing travel time and congestion.
Overall, QBSolv is a powerful tool for solving a wide range of optimization problems in real-world applications.
Its ability to efficiently find optimal solutions to complex problems makes it an invaluable resource for businesses, researchers, and other organizations seeking to optimize their processes and improve their outcomes.
Final Words – Implementing DWave’s QBsolve in Python
In the above blog, we have learned How To Implement Dwave Qbsolve in Python.
The process is not so tedious and lengthy as you just have to install D-Wave ocean SDK, import necessary modules, connect to D-wave Quantum Computer, define a binary quadratic model, and then create an instance of QBSolv class.
Constructing a correct model will be the most tedious task of the work.
If you need any help or consultation regarding Python Development, feel free to contact the Top-Notch Python Developers from APPWRK!
Related Links:
About author
Whether you are planning a start-up or want to enhance your existing business, APPWRK is a one-stop solution to satisfy your goals and expectations. We have action-oriented experience in UI/UX, Mobile, and Web App development. Also, you can knock on our door for Quality Assurance and Digital Marketing services.
Book A Consultation Now!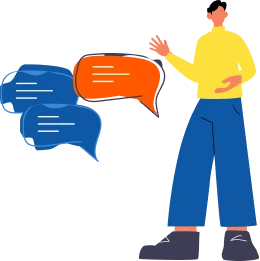