ReactJS Environment Variables: Developer’s Guide [2024]
Hi there, tech enthusiasts! Today, we’re going to dive into a topic that’s often overlooked but tremendously important when working with modern JavaScript frameworks: ReactJS environment variables. An environment variable is a dynamic value, loaded into the application’s context from the environment in which it runs.
Now, let’s shift our focus to ReactJS, a game-changer in the world of web development. ReactJS, an open-source JavaScript library, has been making waves since its inception, owing to its versatility, efficiency, and intuitive nature. It’s the go-to choice for building user interfaces, especially for single-page applications. You’re likely to find it anywhere you see dynamic content that changes without the page reloading.
But here’s the twist: how to use environment variables in ReactJS? As a React developer, you’d want to keep sensitive information away from the prying eyes of the public. That’s where the concept of environment variables in ReactJS comes into play.
ReactJS has a built-in mechanism for accessing environment variables in your application, which helps to ensure your application’s security while also allowing it to behave differently based on the environment it’s in (like development, testing, or production). Using .env files in your React project and accessing these variables through process.env is the standard approach.
So, whether you’re working on a create-react-app project or a custom setup, understanding how to use React env variables can be a game-changer. Ready to dive in? Stay tuned as we unravel the mystery behind ReactJS env variables and how to effectively use them in your projects.
Table Of Contents
What are Environment Variables?
Environment variables are a fundamental component of development in ReactJS. They are variables that are defined outside of your application and can be used within your application. Consider them as invisible hands, delivering essential information to your applications in a secure, efficient, and organized manner.
Why are environmental variables so crucial in the development process?
Let’s say you have an API key that you use throughout your ReactJS application. You wouldn’t want this sensitive piece of information to be visible to everyone, right? That’s where environmental variables come into play. They allow you to securely store such sensitive data and use it within your application without exposing it.
Types of ReactJS ENV Variables
Environment variables in ReactJS can be divided into two main types: system-defined and user-defined.
1. System-defined Environment Variables
System-defined environment variables are pre-set into your system. For instance, PATH or HOME are system-defined environment variables that tell you the system path and the home directory, respectively. You can consider them as the “default settings” of your system, guiding your applications on where to look for certain things.
2. User-defined environment variables
User-defined environment variables are, as the name suggests, defined by the user. They could be anything from your application’s port number to an API key. They’re like your personal Post-it notes, reminding your application of specific values.
Why Do You Need Environment Variables?
In the dynamic sphere of web development, particularly when working with ReactJS, the need for environment variables is as essential as the air we breathe. So why are these invisible, yet substantial elements so crucial? Let’s dive deep into this!
1. The Secret Keepers of Sensitive Information
Environment variables act like confidential agents in the realm of web development. When you’re working with sensitive data such as API keys or database credentials, you need to keep this information secure. Environment variables in ReactJS allow you to safeguard these vital details, letting you use them within your application without exposing them in your code.
2. Promoting a Clean and Organized Codebase
Imagine a world where your code is not just efficient, but also neat and well-organized. Sounds like a dream, doesn’t it? Well, environment variables turn this dream into reality. They assist in maintaining a clear demarcation between configuration and application logic, leading to a codebase that’s easier to manage, read, and debug. This cleanliness contributes to an efficient development process.
3. Seamless Transition Between Environments
One of the shining aspects of ReactJS environment variables is their ability to facilitate smooth transitions between different environments, such as development, testing, and production. Each environment might have its unique configurations, managed conveniently through the .env files specific to each one. This feature brings adaptability right to your doorstep.
4. The Power of Customization
User-defined environment variables are like the icing on the cake. They allow for a level of customization that truly personalizes your application. You can define these variables according to your needs, making them the perfect fit for your application, much like a tailor-made suit.
How to Set up the .env file Reactjs?
Setting up the environment variables in a ReactJS application can be a game-changer! It is a powerful and secure way to manage your project’s configurations, especially when dealing with varying environments like development, testing, and production. Let’s roll up our sleeves and dive into setting up and how to use .env in React!
Creating Your .env File
The first step on this journey is creating a new file in your project’s root directory.
- Open your root project directory. This is where your package.json file resides.
- Create a new file and name it .env.
This file is your golden ticket to managing your environment-specific configurations. But hold on a minute! ReactJS insists on a specific naming convention for environment variables to be recognized.
Naming Conventions: The Key to ReactJS Environment Variables
In the realm of ReactJS, each environment variable must start with the prefix REACT_APP_, followed by the variable name. This prefix is a non-negotiable requirement for React to acknowledge these variables during the build process.
For instance, if you need to store the URL for your API, the environment variable could look something like REACT_APP_API_BASE_URL.
How to Define Variables in the .env File
Defining your variables in the .env file is as easy as pie. Simply open your file and start declaring your variables. An example would be:
REACT_APP_API_BASE_URL=https://api.example.com/
REACT_APP_SECRET_KEY=your-secret-key
But remember, with great power comes great responsibility! Keep your .env file away from prying eyes by not committing it to your version control system. This can be achieved by adding .env to your .gitignore file. Safeguarding your sensitive data has never been so easy!
How to use Environment Variables in ReactJS
Once you’ve defined your environment variables, using them in your React application is a breeze. They can be accessed within your React code using process.env.
Here’s an example:
const apiUrl = process.env.REACT_APP_API_BASE_URL;
console.log(`API Base URL: ${apiUrl}`);
Implementing Environment Variables in a React Component
Let’s see this in action within a React component. Imagine you have a component that fetches data from an API. You’ve stored your API key in your .env file as REACT_APP_API_KEY. Here’s how you could use it:
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const MyComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await axios.get(`https://myapi.com/data?api_key=${process.env.REACT_APP_API_KEY}`);
setData(response.data);
};
fetchData();
}, []);
return (
// Render your data here
);
};
export default MyComponent;
As you can see, process.env.REACT_APP_API_KEY was used to include the API key in the API request, keeping it secure and out of the public eye.
Handling Different Environments
While the .env file is excellent for managing environment variables, what if you want to define different variables for different environments like development, testing, and production?
React got you covered! You can create environment-specific .env files:
- .env: Default
- .env.local: Local overrides. This file is loaded for all environments except test.
- .env.development, .env.test, .env.production: Environment-specific settings.
- .env.development.local, .env.test.local, .env.production.local: Local overrides of environment-specific settings.
Files on the lower (later) lines have more priority than those above.
// .env.production
REACT_APP_API_BASE_URL=https://api.production.com/
// .env.development
REACT_APP_API_BASE_URL=https://api.development.com/
In your application, when you access process.env.REACT_APP_API_BASE_URL, it will automatically pick the correct URL based on the environment your app is running in.
The best approach to using reactjs env file
Adopting the best practices for using .env files in ReactJS can significantly enhance your coding efficiency and the quality of your codebase.
1. Mapping Environment Variables to Readable Names
To create a well-organized and maintainable ReactJS application, mapping environment variables to readable names is a great practice to adopt. This involves giving your environment variables descriptive names that clearly illustrate their purpose.
For instance, if you’re using an API key for a weather application, instead of naming your variable something like REACT_APP_API_KEY, opt for a more specific and readable name such as REACT_APP_WEATHER_API_KEY. This not only enhances readability but also helps in debugging and maintaining the code in the long run.
Let’s consider an example:
// Instead of this
const apiKey = process.env.REACT_APP_API_KEY;
// Use this
const weatherApiKey = process.env.REACT_APP_WEATHER_API_KEY;
As you can see, the latter example clearly indicates the purpose of the API key, making it easier for anyone to read the code.
2. Avoid Adding Strings or Space Characters
Another best practice when using .env files in ReactJS is to avoid adding strings or space characters in your variable values. The values in .env files are treated as strings, so if you wrap your values in quotes or have spaces in your values, they will be included in the actual value. This could lead to unexpected results and bugs that are hard to trace.
For example, consider the following .env file:
// Avoid this
REACT_APP_API_KEY="12345 "
// Do this instead
REACT_APP_API_KEY=12345
In the first example, the API key will have a trailing space, which might cause authentication to fail when using the key. The second example, on the other hand, is the correct way to define environment variables.
Wrapping Up on Environment Variables in ReactJS
Over the course of this discussion, we’ve embarked on a journey exploring the realm of environment variables in ReactJS. Like hidden treasures, they enrich our applications by adding an extra layer of security, efficiency, and adaptability.
Environment variables serve as the guardians of sensitive information, providing a secure haven for data like API keys and database credentials. They help maintain a pristine codebase, promoting neatness and organization. With their help, you can smoothly transition between different environments, making your application versatile and adaptable to the ebb and flow of development, testing, and production environments. They also offer a degree of customization, allowing you to tailor-fit your application to your specific needs.
However, if you find yourself needing a little extra help or expertise, don’t hesitate to contact us. APPWRK IT Solutions has a team of proficient ReactJS Developers ready to assist you in achieving your project goals. Our skilled and experienced team of React developers is always available to offer reliable and efficient support for all your React needs. Whether you’re struggling with performance issues, scalability concerns, or any other aspect of your React application, we have the expertise and know-how to help you overcome any obstacles. Get in touch with us today to learn more about ReactJS development services and experience the benefits of working with expert ReactJS developers!
FAQs
To use environment variables in a ReactJS project, you first need to define them in a .env file located in your project’s root directory. Each variable is defined in the format REACT_APP_VariableName=VariableValue. You can then access these variables in your code using process.env.REACT_APP_VariableName.
You can create a .env file directly in your project’s root directory. This is the same directory where your package.json file is located. You simply create a new file, name it .env, and start defining your variables in the format REACT_APP_VariableName=VariableValue.
Yes, ReactJS allows you to use different .env files for different environments. For example, you can create a .env.development file for your development environment and a .env.production file for your production environment. The correct .env file is used based on the value of the NODE_ENV variable.
You can get the value of an environment variable in your ReactJS code using process.env.REACT_APP_VariableName. Here, VariableName is the name of your variable. Remember that all user-defined variables in a ReactJS project must start with REACT_APP_ to be embedded in your build.
About author
Whether you are planning a start-up or want to enhance your existing business, APPWRK is a one-stop solution to satisfy your goals and expectations. We have action-oriented experience in UI/UX, Mobile, and Web App development. Also, you can knock on our door for Quality Assurance and Digital Marketing services.
Book A Consultation Now!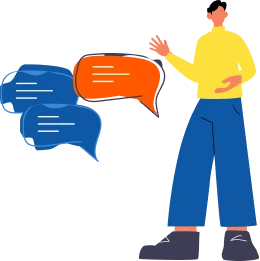